Speech Bubbles
Conversation Displays can exist as standalone objects in a scene, which allows us to set up speech bubbles, also known as World Space Conversation Displays.
Free-floating Converation Displays typically need to make use of a Screen Space Display in order to handle the timing and transition of lines of dialogoue and in case dialogue options need to be shown to the player. Because of this, if you decide to use the Speech Bubble presets included with EasyTalk, you still need to have a Screen Space Dialogue UI in your scene, even if the Screen Space UI itself is never shown to the player. If you want to create a speech bubble which works completely independently of a Screen Space Dialogue Display, read the section about Independent Speech Bubbles.
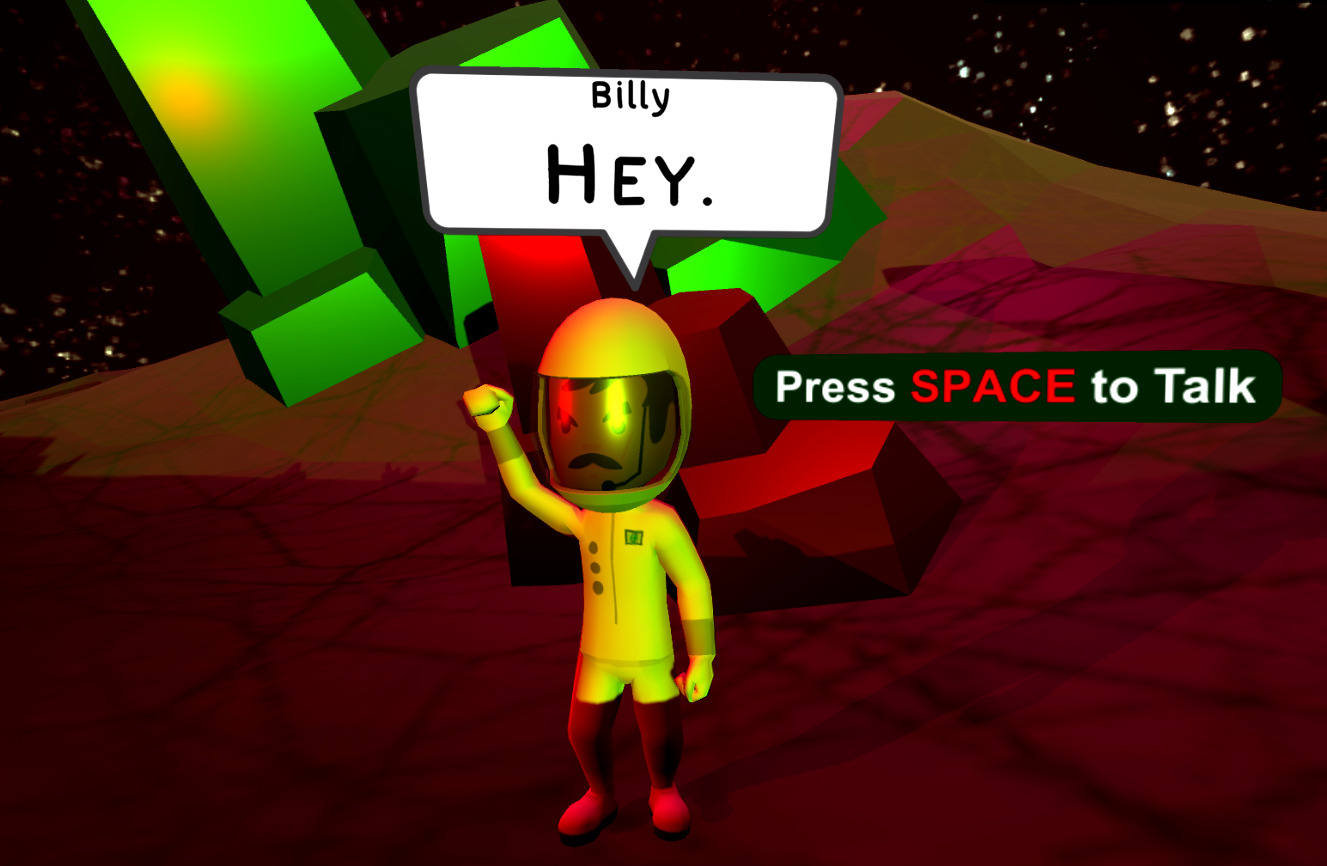
There are some prefabs for speech bubbles in the 'Prefabs/Dialogue Displays/Speech Bubbles/' folder which you can drag and drop into your scene, or onto your characters.
In order to use speech bubbles, you should do one of the following:
- Set the Conversation Display of your character's Dialogue Controller to the Speech Bubble.
- Set the "Switch Convo Display on Character Change" setting to 'true' on your Dialogue Display (under General Settings). This will make the Dialogue Display automatically switch to whichever Conversation Display (Speech Bubble) has a Display ID matching your character's name (set in Conversation Nodes).
- Use [target] tags in your conversation text to target a specific Conversation Display (Speech Bubble).
- Manually change the Conversation Display being used by your Dialogue Display as needed via code.
When using [target] tags or automatic convo display switching based on character name, make sure you set the Display ID of your Speech Bubble / Conversation Display to the name of your character that uses the speech bubble.
Independent Speech Bubbles
If you want, you can create a speech bubble which operates completely independently of a Dialogue Display, such as a Screen-Space Display. The easiest way to do so is:
- Add a Dialogue Controller (or Area Dialogue Controller) to your character.
- Set the Dialogue Controller's 'Use Dialogue Display' property to unchecked, or false.
- Create a subclass of DialogueListener, add a component of the same type to your character somewhere, then add the component to the Dialogue Controller's 'Dialogue Listeners' property.
- Add a world-space canvas UI to the scene, such as above a character's head. (Note: In the example provided here, it should contain a Text component for displaying lines of dialogue).
- Set the Dialogue Controller and Text object on your custom component.
Whenever PlayDialogue() is called on the Dialogue Controller, it will call methods on your Dialogue Listener component which can then update the text as lines of dialogue are processed by the controller.
As an example, you might make a class called SpeechBubble which looks like this:
using UnityEngine.UI;
using EasyTalk.Controller;
//Create a subclass of DialogueListener so we can override methods called by the Dialogue Controller.
public class SpeechBubble : DialogueListener
{
//The Dialogue Controller which will control the speech bubble
[SerializeField] private DialogueController dialogueController;
//The Text component which we will display lines of dialogue on
[SerializeField] private Text dialogueText;
//The amount of time (in seconds) to show each line of dialogue before moving on to the next one
[SerializeField] private float timeBetweenLines = 3.0f;
public void OnDialogueEntered(string entryID)
{
this.SetActive(true);
}
public override void OnDialogueExited(string exitID)
{
this.SetActive(false);
}
public override void OnDisplayLine(ConversationLine line)
{
dialogueText.text = line.Text;
StartCoroutine(WaitToContinue);
}
public IEnumerator WaitToContinue()
{
yield return new WaitForSeconds(timeBetweenLines);
dialogueController.Continue();
}
}
Then just add a SpeechBubble component to your character and set it up as described and you have an independently working speech bubble! 😄
If you use the approach in this example, be aware that it will not support handling dialogue options by default, so make sure that your controller either uses a Dialogue asset without option nodes encountered during dialogue playback, or handle the nodes by overriding the OnDisplayOptions() method and calling ChooseOption() on the Dialogue Controller some time after options are displayed, otherwise the Dialogue Controller will get stuck during dialogue playback.