Dialogue Controllers
Dialogue Controllers are used to play dialogue and process Dialogue Assets, keeping track of the current state of a conversation and determining where to go next.
EasyTalk provides a lot of flexibility with how you can set up your project, but in many cases, it makes the most sense to have a single Dialogue Controller for each character.
Controllers send signals to registered Dialogue Listeners (such as Dialogue Displays) to let them know what's going on in a conversation, such as when the current line of dialogue changes, or when a character change occurs, or when options are supposed to be presented to the player.
It's up to Dialogue Displays and other components to signal the controller back to let it know when to continue or when an option is chosen.
Playing Dialogue
When you want to play dialogue, you just call PlayDialogue() on a controller with the dialogue you want to play:
using EasyTalk.Controller;
...
void Start()
{
//Retrieve the dialogue controller component from the current GameObject
DialogueController controller = GetComponent<DialogueController>();
//Start dialogue playback.
controller.PlayDialogue();
}
You can also provide an entry point ID to the PlayDialogue() method if you have multiple Entry nodes in your dialogue and you want to start from a certain point:
using EasyTalk.Controller;
...
void Start()
{
//Retrieve the dialogue controller component from the current GameObject
DialogueController controller = GetComponent<DialogueController>();
//Start dialogue playback at the Entry node which has the ID of "intro"
controller.PlayDialogue("intro");
}
If you haven't assigned a Dialogue Display to the controller, it will automatically search for a Dialogue Display in the scene and use the first one found whenever you call PlayDialogue().
If you need to switch which Dialogue Asset a controller is using during gameplay, you can call the ChangeDialogue() method.
Controller Settings
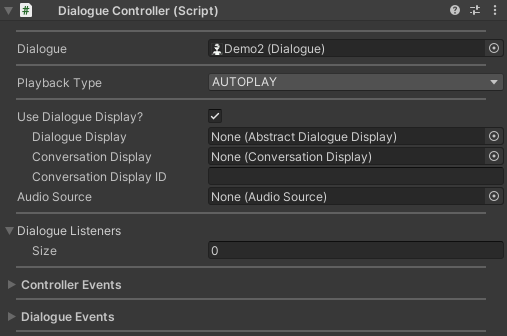
Setting | Description |
---|---|
Dialogue | The Dialogue Asset containing the dialogue or logic which the controller will use. |
Playback Type | Determines how dialogue should be played on the controller, either waiting for player input to continue to the next line of dialogue, or automatically proceeding based on a timer (or the length of audio for a line). |
Dialogue Display | (Optional) Tells the controller which dialogue display it should use when PlayDialogue() is called. If this isn't set, it will search for one in the scene. |
Conversation Display | (Optional) The conversation display to use for displaying dialogue for this controller. If left empty, the Dialogue Display will use its currently set conversation display. |
Audio Source | (Optional) The audio source to use when playing audio for lines of dialogue. If left empty, the Dialogue Display will attempt to use an audio source of its own instead. |
Dialogue Listeners | Dialogue Listeners to call as events happen during dialogue playback. Controllers automatically register and unregister Dialogue Displays as needed, but you can add your own Dialogue Listeners here if you want to implement custom logic that responds to dialogue playback. |
Dialogue Listeners
Dialogue Listeners can be added to this list and their relevant methods will be called as events take place during dialogue playback. By creating your own Dialogue Listener extension class you can create more complex systems which respond to dialogue events.
Controller Events
Event Name | Description |
---|---|
On Play | Triggered whenever dialogue playback starts. |
On Stop | Triggered whenever dialogue playback stops. |
Dialogue Events
Event Name | Description |
---|---|
On Continue | Called whenever dialogue playback continues to the next line of dialogue, or in some cases, the next node. |
On Display Options | Called whenever dialogue options are to be presented to the player. |
On Option Chosen | Called whenever a dialogue option has been chosen/finalized. |
On Display Line | Called whenever a line of dialogue is to be displayed. |
On Dialogue Entered | Called whenever dialogue playback begins. |
On Dialogue Exited | Called whenever dialogue playback ends. |
On Exit Completed | Called one frame after dialogue playback ends. |
On Story | Called whenever a story node is encountered during dialogue playback. |
On Variable Updated | Called whenever a dialogue variable's value is changed. |
On Character Changed | Called whenever a character change is detected. |
On Audio Started | Called whenever audio playback starts for a line of dialogue. |
On Audio Completed | Called whenever audio playback stops or finishes for a line of dialogue. |
On Activate Key | Called whenever a line of dialogue is being processed. |
On Wait | Called whenever a Wait node is encountered during dialogue playback. |
On Conversation Ending | Called whenever dialogue playback reaches the last line of dialogue in a Conversation node. |
On Node Changed | Called whenever dialogue playback moves from one node to another. |
On Pause | Called whenever a Pause node is encountered during dialogue playback. |